HTML to PDF in under 2 seconds
Our API makes it easy to export high-quality PDF versions of HTML documents from raw code or URLs. There's no need to worry about CSS properties, outdated libraries or conversion errors.
See Our GuidesGenerate beautiful previews of any website
Equip your product with the ability to turn visual content into downloadable images. Grab up-to-date screenshots from any website to generate beautiful previews and customize image output to suit your needs. All it takes is one simple API call.
See Our GuidesAutomate blog banners for social networks
Generate impactful Open Graph images to share your content on social networks easily. Use custom templates and provide variable parameters to automatically integrate banners into your CMS.
See Our GuidesStore your template at PDFShift and increase conversion speed
Store your template and take advantage of our powerful template engine to convert your documents even faster. Storing your templates at PDFShift will allow you to generate documents on the fly with your customer in a secure way. It will allow you to automatically build banner images for your blog posts securely within your blog engine (Wordpress, Next, Nuxt).
See Our GuidesSecurely store your content in your S3 Bucket
Our API makes it easy to export high-quality PDF versions of HTML documents from raw code or URLs. There's no need to worry about CSS properties, outdated libraries or conversion errors.
See Our GuidesPowerful features for high-fidelity documents
Render pages beautifully with full CSS Support
Our powerful engine supports all the latest CSS features, including custom web fonts, flexbox, grid layouts, and responsive design.
Tailor documents to suit your needs
Customize headers and footers, inject custom CSS and Javascript, and wait for custom elements on the page so they turn out perfect every time.
Convert documents securely
Beam your raw HTML directly to PDFShift for completely secure conversions ‐ perfect for handling sensitive data.
Play nice with third-party libraries
PDFShift works great with external libraries such as Tailwind, Bootstrap, Bulma, Skeleton, …. You can also generate beautiful charts with javascript libraries or maps using Google Map or OpenStreetMap.
Generate documents in the blink of an eye
Use PDFShift's powerful webhook system to quickly generate thousands of documents with minimal wait times. Need more? Invoke our parallel conversion system to convert many documents with a single request for results in milliseconds.
Guaranteed privacy with HIPAA compliance
PDFShift's conversion technology is fully HIPAA compliant, making it a great choice for the healthcare industry. We don't store your documents (unless specifically requested in the API), so we can ensure the privacy of your data.
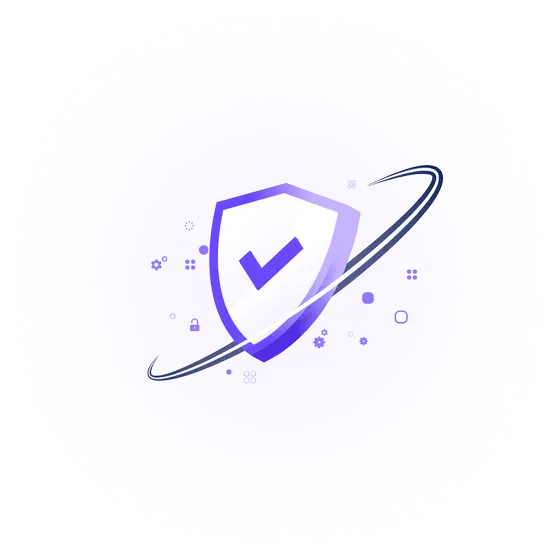
Pricing
Start for free with up to 50 credits per month
Upgrade based on your needs
Select your monthly conversion needs
Free plan
- CSS & Javascript injection
- Advanced header & footer
- Encryption and Watermark
- No file size limit
- AWS S3 delivery
- Parallel & Asynchronous responses
* We count 1 credit per 5Mb. For example, a 14Mb PDF will consume 3 credits.
Frequently Asked Questions
- 1Can I try PDFShift for free?
- 2What can I do with PDFShift?
- 3Can I try PDFShift without creating an account?
- 4How do you ensure high-quality conversions and service uptime?
- 5I use Amazon S3 storage for my converted documents — how is my data privacy maintained?
- 6How do credits work, and how are conversions counted?
- 7What happens if I exceed my API request volume?
- 8How does your overage system work?
- 9What if I have custom requirements?
Our dedicated FAQ page contains answers to many more questions.
If you can't find what you're looking for, feel free to reach out to us via email, and we'll be happy to answer to all your questions.
Trusted and loved
around the world
Hundred of users shared their love of PDFShift